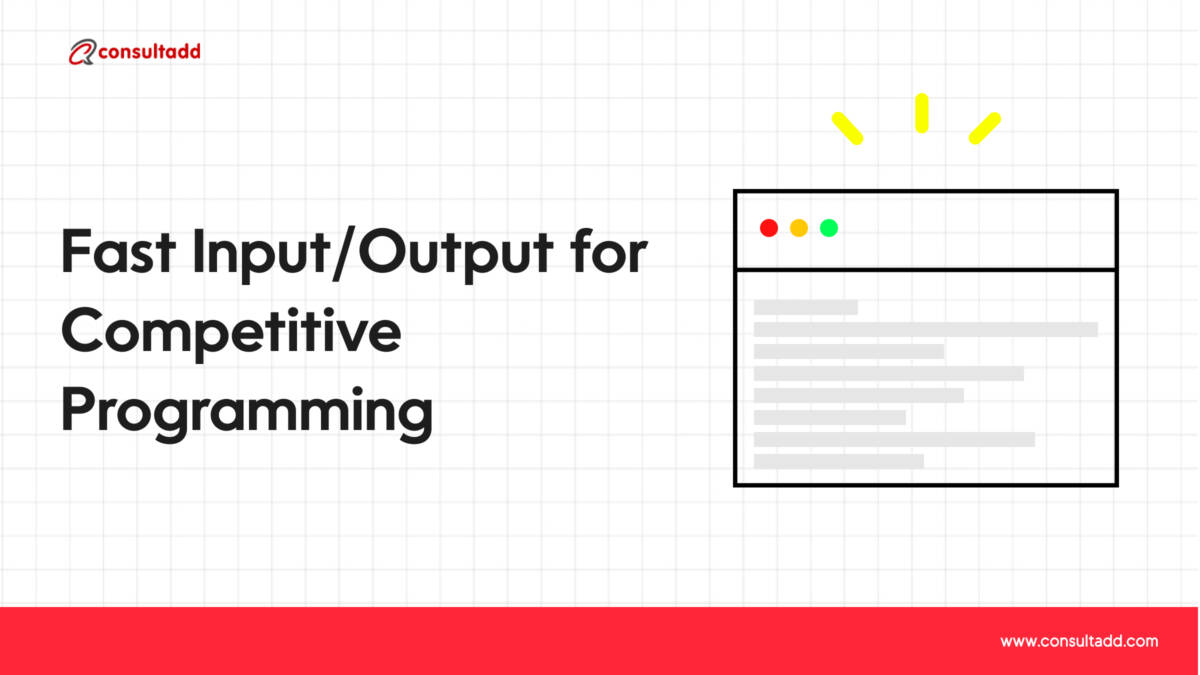
In order to save critical time in competitive programming, it is crucial to read input as quickly as possible.
You’ve probably come across a number of problem statements that include the phrase “Warning: Large I/O data, be careful with certain languages (but most should be OK if the algorithm is adequately developed)” Utilizing fast Input/Output techniques is the solution to such issues.
For quick input and output, scanf/printf is frequently advised in preference to cin/cout. However, by inserting the following two lines in your main() function, you may still utilize cin/cout and obtain the same speed as scanf/printf. It is advised to substitute cout “n”; for cout endl;. Endl forces an unneeded flushing stream, which makes it slower.
ios_base:sync_with_stdio(false);
If it is called prior to the program’s first input or output operation, it turns on or off the synchronization of all standard C++ streams with their equivalent standard C streams. This synchronization can be avoided by adding ios base::sync with stdio (false); before any I/O action (which is true by default). It is a static member of the std::ios base function.
cin.tie(NULL);
The procedure tie() merely ensures that std::cout flushes before std::int. cin will take command. This is advantageous for interactive console programmes that necessitate continuous console updates but slows down the program for heavy I/O. The NULL portion merely returns a pointer to NULL.
Additionally, you just need to use one include to include the standard template library (STL):
#include <bits/stdc++.h>
Thus, your model for competitive programming might be as follows:
#include <bits/stdc++.h>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
return 0;
}
It is advised to substitute cout “n”; for cout endl;. Because endl demands a flushing stream, which is typically unnecessary, it takes longer. (You wouldn’t need to flush when writing a million lines of data; you would need to flush when creating, say, an interactive progress bar.) Instead of writing endl, use ‘n.
On the issue INTEST – Enormous Input Teston SPOJ, we can test our input and output techniques. We would recommend that you fix the problem first before continuing to read. Thus, fast Input/Output can let you have the edge over competitive programming.